Take Screenshots with python Tkinter
In this tutorial, we’ll guide you through the process of how to screenshot on windows by creating a Python application using the Tkinter library. It allows you to capture screenshots at regular intervals simply by clicking a button. This simple Tkinter application provides a user-friendly interface for configuring various settings and controlling the screenshot capture process. Follow the steps below to set up and use the provided code for the take_screenshot_loop
application.
Step 1: Install Required Libraries
Before you start, ensure you have Python installed on your system. Additionally, install the required libraries using the following command in your terminal or command prompt:
pip install pillow tkinter
Step 2: How to screenshot on windows: Saving the Code
Copy the provided code and save it in a file, for example, screenshot_looper.py
.
import time
import os
from PIL import ImageGrab
import tkinter as tk
from tkinter import ttk, messagebox
class TakeScreenshots:
def __init__(self, master):
self.master = master
self.master.title("Take Screenshot Looper")
self.master.geometry('300x450')
self.create_widgets()
def create_widgets(self):
ttk.Label(self.master, text="Object File Name:").pack(pady=5)
self.mob_entry = ttk.Entry(self.master)
self.mob_entry.insert(0, "example")
self.mob_entry.pack(pady=5)
ttk.Label(self.master, text="Monitor (left, top, right, bottom):").pack(pady=5)
self.monitor_entry = ttk.Entry(self.master)
self.monitor_entry.insert(0, "40, 0, 800, 640")
self.monitor_entry.pack(pady=5)
ttk.Label(self.master, text="Display Time (seconds):").pack(pady=5)
self.display_time_entry = ttk.Entry(self.master)
self.display_time_entry.insert(0, "0.5")
self.display_time_entry.pack(pady=5)
ttk.Label(self.master, text="Initial Image Index:").pack(pady=5)
self.img_entry = ttk.Entry(self.master)
self.img_entry.insert(0, "0")
self.img_entry.pack(pady=5)
ttk.Label(self.master, text="Directory:").pack(pady=5)
self.directory_entry = ttk.Entry(self.master)
self.directory_entry.insert(0, "./datasets/osrs/")
self.directory_entry.pack(pady=5)
self.status_label = ttk.Label(self.master, text="")
self.status_label.pack(pady=10)
ttk.Button(self.master, text="Start", command=self.start_capture).pack(pady=5)
ttk.Button(self.master, text="Stop", command=self.stop_capture, state=tk.DISABLED).pack(pady=5)
self.img = 0
self.is_capturing = False
def ensure_dir(self):
self.directory = self.directory_entry.get()
if not os.path.exists(self.directory):
os.makedirs(self.directory)
def start_capture(self):
self.status_label.config(text="Running", foreground='#8fce00')
self.master.iconify()
time.sleep(0.2)
self.mob = self.mob_entry.get()
monitor_values = list(map(int, self.monitor_entry.get().split(',')))
self.monitor = tuple(monitor_values)
self.display_time = float(self.display_time_entry.get())
self.img = int(self.img_entry.get())
self.directory = self.directory_entry.get()
self.is_capturing = True
self.start_button.config(state=tk.DISABLED)
self.stop_button.config(state=tk.NORMAL)
self.capture_images()
def stop_capture(self):
self.status_label.config(text="Stopped", foreground='#f44336')
self.is_capturing = False
self.start_button.config(state=tk.NORMAL)
self.stop_button.config(state=tk.DISABLED)
def capture_images(self):
while self.is_capturing:
try:
im = ImageGrab.grab(bbox=self.monitor)
im.save(os.path.join(self.directory, f'{self.mob}_{self.img}.jpg'))
self.img += 1
time.sleep(self.display_time)
self.master.update()
except Exception as e:
messagebox.showerror("Error", str(e))
self.is_capturing = False
if __name__ == "__main__":
root = tk.Tk()
app = TakeScreenshots(root)
root.mainloop()
Step 3: Run the Application
Open a terminal or command prompt, navigate to the directory containing the saved script (screenshot_looper.py
), and run the following command:
python screenshot_looper.py
Step 4: Configure Settings
Once the application window opens, you’ll see several Entry widgets to input the following settings:
- Object File Name: The prefix for the screenshot filenames.
- Monitor (left, top, right, bottom): Define the area on the screen to capture in the format left, top, right, bottom.
- Display Time (seconds): Set the time interval between each screenshot.
- Initial Image Index: The starting index for the screenshot filenames.
- Directory: The directory where screenshots will be saved.
Step 5: Start and Stop Capture
Click the “Start” button, this is how to screenshot on windows which is to initiate the screenshot capture process with the specified settings. To stop capturing screenshots, click the “Stop” button.
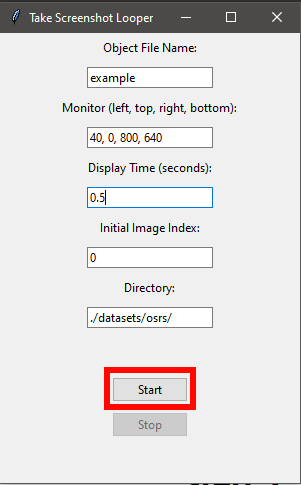
Step 6: Review Captured Screenshots
Navigate to the specified directory to find the captured screenshots. The filenames will be in the format {object_file_name}_{image_index}.jpg
.
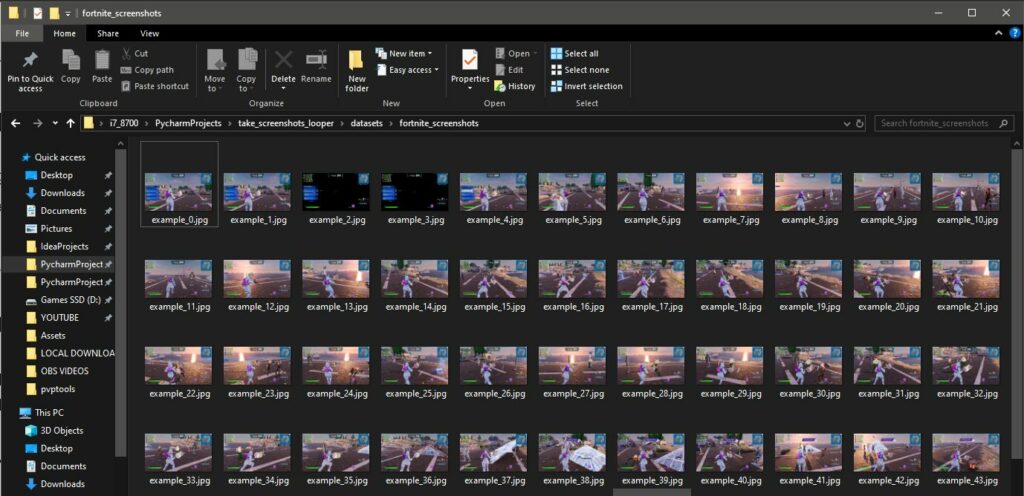
Notes
- If the specified directory does not exist, the application will create it.
- If an error occurs during the capture process, an error message will be displayed.
Conclusion
This Tkinter application provides a convenient way on how to screenshot on windows by capturing screenshots at regular intervals, making it useful for various tasks such as creating datasets or monitoring visual changes over time. Customize the settings based on your specific requirements, and enjoy the ease of capturing screenshots with this user-friendly interface. Whether you’re a developer, data scientist, or simply someone who needs periodic screenshots, this application streamlines the process for you.
Want to know how the code works? TBA soon for tutorial on how the elements and functions work in this screenshot tkinker application.
Thanks-a-mundo for the blog.Much thanks again. Much obliged.
Thank you ever so for you blog.Really thank you! Keep writing.
Looking forward to reading more. Great article.Thanks Again. Cool.
I am so grateful for your article.Really thank you! Awesome.
Awesome article post.Really looking forward to read more. Will read on…
Thanks for the blog article.Thanks Again. Great.
Thanks for posting when you have the opportunity,
Fastidious response in return of this query with firm argumentsand telling the whole thing concerning that.
Effectively voiced of course! !custom essays college admission essay writing service phd dissertation writing service
I wanted to thank you for this fantastic read!! I absolutely loved every bit of it. I have got you book marked to look at new stuff you postĂ
Hi there, after reading this awesome post i am too delighted toshare my familiarity here with friends.
Magnificent website. Lots of useful info here. Iâm sending it to a few friends ans also sharing in delicious. And certainly, thanks for your sweat!
Wow, amazing weblog format! How lengthy have you been blogging for? you make running a blog glance easy. The whole look of your web site is magnificent, as neatly as the content material!
Thank you for sharing excellent informations. Your web-site is so cool. I am impressed by the details that youâve on this blog. It reveals how nicely you understand this subject. Bookmarked this web page, will come back for extra articles. You, my pal, ROCK! I found just the info I already searched all over the place and simply could not come across. What a perfect web-site.
Hello there, You have performed a fantastic job. I will certainly digg it and in my opinion suggest to my friends. I am confident they’ll be benefited from this web site.
Thank you so much for giving everyone an exceptionally superb possiblity to read critical reviews from here. It can be very cool and as well , packed with amusement for me and my office colleagues to search your web site minimum thrice every week to find out the newest issues you have. Of course, I’m so at all times amazed considering the impressive techniques you serve. Selected 4 areas in this posting are unquestionably the simplest we have all ever had.
Itâs really a great and useful piece of info. I am glad that you shared this helpful information with us. Please keep us informed like this. Thanks for sharing.
Yesterday, while I was at work, my cousin stole my iPad and tested to see if it can survive a forty foot drop, just so she can be a youtube sensation. My apple ipad is now broken and she has 83 views. I know this is completely off topic but I had to share it with someone!
Good site! I really love how it is simple on my eyes and the data are well written. I am wondering how I might be notified whenever a new post has been made. I have subscribed to your feed which must do the trick! Have a great day!
I cling on to listening to the reports talk about receiving boundless online grant applications so I have been looking around for the finest site to get one. Could you tell me please, where could i acquire some?
Somebody essentially help to make seriously articles I would state. This is the very first time I frequented your website page and thus far? I amazed with the research you made to create this particular publish amazing. Magnificent job!
This is a very good tip particularly to those new to the blogosphere. Short but very precise infoĂ Thanks for sharing this one. A must read article!
Excellent goods from you, man. I’ve be aware your stuff previous to and you’re just too great. I really like what you’ve bought right here, certainly like what you’re stating and the way in which in which you assert it. You make it enjoyable and you continue to care for to keep it smart. I can’t wait to read much more from you. This is really a terrific site.
An fascinating dialogue is value comment. I feel that you need to write extra on this topic, it won’t be a taboo topic but typically people are not sufficient to speak on such topics. To the next. Cheers
I think this is a real great blog post.Really looking forward to read more. Awesome.
I really liked your blog.Really looking forward to read more. Awesome.
Thanks for sharing, this is a fantastic blog article.Much thanks again. Awesome.
Don’t stuff yourself into too compact lingerie, look around and see that you may have any piece of full figure lingerie you desire in your measurement.
Major thanks for the blog.Much thanks again. Great.
Hi, I do think this is a great blog. I stumbledupon it đ I will revisit yet again since I saved as a favorite it. Money and freedom is the best way to change, may you be rich and continue to help others.
Thank you for your blog article.Thanks Again. Awesome.
A round of applause for your blog post.Really looking forward to read more. Keep writing.
Hire essay writer best essay writer companythe best essay writers
Major thankies for the article post.Really looking forward to read more. Great.
wow, awesome blog. Want more.
Wow, great article.Thanks Again. Great.
I don’t even know how I ended up here, but I believed this post used to be good. I don’t recognise who you might be however certainly you’re going to a famous blogger should you are not already đ Cheers!
There as definately a lot to find out about this subject. I love all of the points you made.
ŕ¸ŕ¸ŕ¸ąŕ¸ŕ¸ŕ¸ŕ¸Ľŕ¸ŕ¸˘ŕšŕ¸˛ŕ¸ŕšŕ¸Łŕšŕ¸Ťŕšŕšŕ¸ŕšŕšŕ¸ŕ¸´ŕ¸ ŕšŕ¸ŕ¸ŕ¸ŕ¸ŕ¸Ľŕ¸ŕ¸ŕ¸°ŕ¸ŕ¸˘ŕšŕ¸˛ŕ¸ŕšŕ¸Łŕšŕ¸Ąŕšŕ¸ŕ¸šŕ¸ŕšŕ¸ŕ¸ UFABET ŕ¸ŕšŕ¸˛ŕ¸˘ŕ¸ŕ¸Łŕ¸´ŕ¸ŕ¸ŕšŕ¸˛ŕ¸˘ŕšŕ¸Ąŕšŕ¸˘ŕ¸ąŕšŕ¸ŕ¸Łŕ¸°ŕ¸ŕ¸ŕšŕ¸ŕšŕ¸˛ŕšŕ¸ŕ¸ŕšŕ¸˛ŕ¸˘ ŕšŕ¸ŕšŕšŕ¸ŕ¸ľŕ¸˘ŕ¸ŕ¸ŕ¸Ľŕ¸´ŕ¸ŕ¸Şŕ¸Ąŕ¸ąŕ¸ŕ¸Łŕ¸ŕšŕ¸ŕ¸łŕšŕ¸ŕ¸´ŕ¸ŕšŕ¸ŕšŕ¸ŕ¸˘ŕšŕ¸˛ŕ¸ŕ¸ŕšŕ¸˛ŕ¸˘ŕšŕ¸ŕ¸ąŕ¸ŕ¸ŕ¸˛ŕ¸Şŕ¸´ŕšŕ¸ŕ¸ŕ¸ŕ¸ŕšŕ¸Ľŕ¸ŕš สŕ¸ŕ¸˛ŕ¸˘ŕ¸Şŕ¸¸ŕ¸ŕšŕ¸ŕ¸ľŕ¸§ŕ¸´ŕ¸ŕ¸ŕ¸´ŕ¸§ŕšŕ¸ŕ¸ľŕ¸§ŕ¸´ŕ¸ŕ¸ŕ¸Ľŕ¸šŕšŕ¸ŕ¸łŕšŕ¸ŕ¸´ŕ¸ สรŕšŕ¸˛ŕ¸ŕ¸ŕ¸łŕšŕ¸Łŕ¸Şŕ¸ŕ¸˛ŕ¸˘ŕšŕ¸ŕ¸śŕ¸ŕ¸ŕ¸§ŕ¸Łŕ¸ŕ¸ľŕš UFABET
Remarkable issues here. I’m very glad to peer your post.Thanks a lot and I am having a look forward to contact you.Will you kindly drop me a e-mail?
Hey just wanted to give you a quick heads up and let you know afew of the images aren’t loading correctly.I’m not sure why but I think its a linking issue.I’ve tried it in two different browsers and both show the sameresults.
hydroxychloroquine pills what does hydroxychloroquine treat
Really no matter if someone doesn’t be aware of then its upto other people that they will help, so here it occurs.
Very well written post. It will be supportive to anybody who employess it, including myself. Keep up the good work â for sure i will check out more posts.
Good blog you have got here.. It’s hard to find good quality writing like yours nowadays. I really appreciate individuals like you! Take care!!
An intriguing discussion is definitely worth comment. I think that you need to publish more about this topic, it might not be a taboo subject but generally people don’t speak about these issues. To the next! Best wishes!!
Hi i am kavin, its my first time to commenting anywhere,when i read this post i thought i could also make comment due to this brilliantparagraph.
This is a really good tip particularly to those fresh to the blogosphere.Simple but very precise info… Appreciate your sharing this one.A must read post!my blog â eczema remedies
Hello, all is going perfectly here and ofcourse every one is sharing information, that’s genuinely fine, keep up writing.
Hello mates, fastidious piece of writing and pleasant urging commented here, I am genuinely enjoying by these.
I appreciate you sharing this post. Fantastic.
Heya! I just wanted to ask if you ever have any trouble with hackers?My last blog (wordpress) was hacked and I ended up losing several weeks of hard work due to no backup.Do you have any methods to protect against hackers?
Very informative blog post.Really looking forward to read more. Much obliged.
Does anyone know if I am able to purchase JUSTKRATOM Red Maeng Da Kratom Capsules (justkratomstore.com) from American Pure Vapor, 1830 NE Pine Island Road #110, Cape Coral, FL, 33909?
Hey! Do you use Twitter? I’d like to follow you if that wouldbe okay. I’m absolutely enjoying your blog and look forward to new updates.
pretty practical stuff, overall I imagine this is worthy of a bookmark, thanks
I want to to thank you for this good read!! I definitely enjoyed every little bit of it. I have got you saved as a favorite to check out new things you post…
These are truly wonderful ideas in on the topic of blogging.You have touched some nice points here. Any way keep up wrinting.
Very neat blog article.Much thanks again. Fantastic.
Hey! This post could not be written any better! Reading through this post reminds me of my good old room mate! He always kept talking about this. I will forward this post to him. Pretty sure he will have a good read. Thanks for sharing!
Some really superb info , Gladiolus I detected this. “It’s not what you are that holds you back, it’s what you think you are not.” by Denis Watley.
Just Browsing While I was surfing today I saw a great article about
I truly appreciate this post. I’ve been looking everywhere for this!Thank goodness I found it on Bing. You have made my day!Thanks again!Also visit my blog â cannabis vodka
Say, you got a nice article post. Thanks Again. Keep writing. Joscelin Barri Grayce
I truly appreciate this article.Really looking forward to read more.
Thanks so much for the article post.Much thanks again. Want more.
Thanks again for the article post. Great.
This is one awesome blog article. Will read on…
Hi, I do believe this is an excellent blog. I stumbledupon it đ I am going to revisit once again since I bookmarked it. Money and freedom is the greatest way to change, may you be rich and continue to help other people.
A round of applause for your post.Really thank you! Fantastic.
It’s hard to come by well-informed people in this particular subject, however,you seem like you know what you’re talking about! Thanks
Thank you for sharing your thoughts. I truly appreciate your effortsand I will be waiting for your further write ups thanks once again.
A round of applause for your post.Much thanks again. Really Cool.
Great, thanks for sharing this blog article.Really thank you! Fantastic.
I truly appreciate this blog.Much thanks again. Will read on…
What’s up, I wish for to subscribe for this blog to take newest updates, thus where can i do it pleasehelp out.Here is my blog post :: Niagara XL Review
Great blog article.Much thanks again. Will read on…
It is really great to see that you are still creating great articles after all this time. Good job on this article! Interesting content. Thumbs up!
Really informative post.Really looking forward to read more. Cool.
I really enjoy the article post.Thanks Again. Really Great.
Good Morning everyone , can anyone advise where I can purchase Jam Monster CBD?
Nice post. I used to be checking constantly this blog and I am inspired!Extremely helpful info specially the closing part đ I handle suchinfo much. I was looking for this certain info for a long time.Thank you and good luck.
What’s up, all the time i used to check blog posts here in the early hours in the break of day, for the reason that i like to learn more and more.
Hey! Would you mind if I share your blog with my zynga group?There’s a lot of folks that I think would really appreciate your content.Please let me know. Thanks
Very good blog article.Much thanks again. Cool.
It’s hard to find knowledgeable people on this subject, but you sound like you know what you’re talking about!Thanks
Hi my friend! I wish to say that this article is amazing, great written and come with approximately allsignificant infos. I’d like to look more posts like this .
procom smart spaces radiation gave me cancer
Pretty component to content. I simply stumbled upon yourblog and in accession capital to say that I acquire in fact enjoyed account your blog posts.Anyway I’ll be subscribing in your augment or even I success you access persistently fast.
I’ll right away grasp your rss as I can not in finding youre-mail subscription hyperlink or e-newsletter service.Do you’ve any? Kindly permit me know so that I could subscribe.Thanks.
weight loss pills naltrexone naltrexone injection pdf revia moa
how much is plaquenil secdn â hydroxychloroquine sulfate tablet
phd dissertation search what is dissertation
zithromax classification augmentin vomiting cephalexin cap
I am a big fan of this blog. I’ll return to read more soon. Thanks for creating it.
prednisone cream over the counter â prednisplus.com prednisone brand name
There’s certainly a great deal to learn about this subject. I like all of the points you’ve made.
sildenafil starting dosage sildenafil overdose
632875 820335If I need to say something, then absolutely nothing will stop the chatter within 287911
TeamViewer is super easy to arrange and has an strange consumer interfaceFree License Key â Get a Free Activation Codeâ Serial Keylicense key
I will immediately clutch your rss as I can’t find your email subscription hyperlink ornewsletter service. Do you have any? Kindly permit me understand in order that Imay subscribe. Thanks.
I like the helpful info you provide in your articles. I will bookmark your blog and take a look at again right here regularly. I’m reasonably certain I’ll be informed many new stuff proper here! Good luck for the next!
Thank you for the good writeup. It in fact was once a amusement account it.Look advanced to far brought agreeable fromyou! However, how could we keep in touch?
Hi there, just became alert to your blog through Google, andfound that it is really informative. I’m gonna watch outfor brussels. I will be grateful if you continue this in future.Many people will be benefited from your writing.Cheers!
Excellent post. I am going through many of these issues as well..
Thanks for the blog post.Thanks Again. Much obliged.
I do accept as true with all the ideas you’ve offeredfor your post. They are very convincing and can certainly work.Still, the posts are too brief for newbies. Could you please extend them a little from subsequent time?Thanks for the post.
Hello my family member! I wish to say that this article is amazing, great written and include almostall vital infos. I’d like to peer extra posts like this .
I am genuinely delighted to glance at this blog posts which contains plenty of useful facts, thanks for providing these kinds of data.
A fascinating discussion is worth comment. I do think that you ought to write more about this subject, it might not be a taboo subject but typically people do not talk about these issues. To the next! Cheers!!
Does anyone know if I am able to purchase JUSTKRATOM Trainwreck Kratom Capsules (justkratomstore.com) at Avail Vapor, 2116 Wayne Memorial Drive, Unit B, Goldsboro, NC, 27534?
Perform the following to discover more about women before you are left behind.
Muchos Gracias for your blog.
You are a very intelligent individual!Also visit my blog post: Frost Air Conditioning
Thanks for the article post. Fantastic.
Where do you come from? This calculator will show you just how long it’s going to take you to clear your credit card balance if you don’t wake up, face reality, stop paying the bare minimum and start clearing this punitive form of debt.
Looking forward to reading more. Great post.Much thanks again. Really Great.
Very informative article post.Thanks Again. Great.
I appreciate you sharing this post.Thanks Again. Want more.
Fantastic article post.Really looking forward to read more. Will read on…
We are searching for some people that might be interested in from working their home on a part-time basis. If you want to earn $100 a day, and you don’t mind developing some short opinions up, this is the perfect opportunity for you!
Fantastic article post.Thanks Again. Much obliged.
It’s actually a great and useful piece of information. I’m happy that you simply shared this helpful info with us. Please stay us up to date like this. Thank you for sharing.
An interesting discussion is worth comment. I do think that you need to write more about this subject matter, it might not be a taboo matter but generally folks don’t talk about such topics. To the next! Cheers!
Major thankies for the article post. Keep writing.
Thank you ever so for you post.Much thanks again. Really Great.
Thank you ever so for you blog article. Much obliged.
That is a very good tip particularly to those fresh tothe blogosphere. Short but very precise information… Manythanks for sharing this one. A must read post!
Im thankful for the article post.Thanks Again. Cool.
I appreciate you sharing this article post.Much thanks again. Cool.
This is one awesome blog article.Thanks Again. Will read on…
I truly appreciate this article.Really looking forward to read more.
It’s in reality a nice and useful piece of information. I am happy thatyou simply shared this useful information with us.Please stay us up to date like this. Thank you for sharing.
Joanna Newsom The Milk Eyed Mender Jon Bon Jovi Blaze Of Glory The Beatles 1967 1970
Utterly indited written content, Really enjoyed looking through.
This is a very good tip especially to those fresh to the blogosphere. Simple but very precise information… Many thanks for sharing this one. A must read post!
I really liked your blog article. Fantastic.
Thanks so much for the article post.Thanks Again. Want more.
We are a group of volunteers and opening a new scheme in our
community. Your web site offered us with helpful information to work on. You’ve done
an impressive process and our entire community will be grateful to you.
Also visit my homepage … nordvpn coupons inspiresensation (t.co)
Exactly what I was searching for, thankyou for putting up.
Awesome blog article.
It’s going to be end of mine day, however before finish I am readingthis wonderful article to increase my experience.
Muchos Gracias for your blog article.Really thank you! Awesome.
Oh my goodness! an amazing article dude. Thanks However I’m experiencing challenge with ur rss . Don’t know why Unable to subscribe to it. Is there anybody getting similar rss drawback? Anyone who is aware of kindly respond. Thnkx
blox unlimited robux and tix roblox robux hack script pastebin how to get
you can check here view of Three Gorges Wonder Travel Blog
Very good post. Really Cool.
I cannot thank you enough for the blog.Really looking forward to read more. Much obliged.
I think this is a real great post.Really thank you!
I cannot thank you enough for the blog article.Much thanks again.
Thank you for your article.Really looking forward to read more. Really Great.
Just bookmarked this blog post as I have actually found it fairly valuable.
Muchos Gracias for your article post. Want more.
This is one awesome article.Really thank you! Fantastic.
I really liked your blog post.Really looking forward to read more. Much obliged.
I really loved this piece of content. I’ll return to read more. Thank you for creating it!
Very good article.Much thanks again. Really Cool.
Muchos Gracias for your article post.Much thanks again. Much obliged.
Thank you ever so for you article.Really looking forward to read more. Want more.
What’s up i am kavin, its my first occasion to commentinganyplace, when i read this paragraph i thoughti could also create comment due to this sensible article.Also visit my blog post :: weight loss results
This is one awesome blog post.Thanks Again.
Muchos Gracias for your blog article.Really thank you! Keep writing.
Thanks for sharing, this is a fantastic blog article.Really looking forward to read more. Keep writing.
A round of applause for your blog.Really thank you! Will read on…
I value the article post.Thanks Again. Really Cool.
Im thankful for the blog article.Really looking forward to read more. Fantastic.
Thank you for your article.Much thanks again. Fantastic.
Im thankful for the article.Really thank you! Will read on…
I value the blog article.Much thanks again. Cool.
Enjoyed every bit of your blog article.
Im obliged for the blog article. Much obliged.
I cannot thank you enough for the blog.Much thanks again. Much obliged.
Thank you for your blog article.Thanks Again. Really Cool.
Thanks a lot for the blog article.Really looking forward to read more. Really Cool.
Major thankies for the article post.Really thank you! Cool.
Say, you got a nice article.Thanks Again. Awesome.
Enjoyed every bit of your post.
Really appreciate you sharing this article.Much thanks again. Will read on…
Thanks for the post.Really looking forward to read more. Really Great.
Thanks for sharing, this is a fantastic article post.Really looking forward to read more. Want more.
I really like and appreciate your blog post.Really thank you! Keep writing.
Wow, great article.Thanks Again. Much obliged.
Appreciate you sharing, great article.Thanks Again. Fantastic.
Very good blog post.Really looking forward to read more. Keep writing.
I really enjoy the post.Much thanks again. Really Great.
Im grateful for the post.Much thanks again. Fantastic.
I truly appreciate this blog article.Thanks Again. Great.
Thank you ever so for you blog.Really thank you! Much obliged.
I appreciate you sharing this article post.Really thank you! Want more.
Very neat blog. Much obliged.
Looking forward to reading more. Great blog article.Much thanks again. Cool.
Say, you got a nice blog post.Really looking forward to read more. Fantastic.
Looking forward to reading more. Great blog article.Really thank you! Really Cool.
Thanks so much for the blog article.Much thanks again. Want more.
Thanks a lot for the article post.Thanks Again. Great.
wow, awesome blog.Thanks Again. Much obliged.
Great, thanks for sharing this blog article.Thanks Again.
Hey, thanks for the post.Really looking forward to read more. Want more.
Very informative post. Cool.
Thanks for the blog article. Really Cool.
Thank you for your article post.Thanks Again. Great.
Thank you ever so for you blog article.Really looking forward to read more. Much obliged.
Thanks a lot for the article. Great.
Very neat article.Really looking forward to read more. Much obliged.
Really informative blog.Thanks Again. Keep writing.
Say, you got a nice blog post.
A round of applause for your blog article.Much thanks again. Want more.
Thanks for sharing, this is a fantastic blog post.Much thanks again.
Muchos Gracias for your blog.Much thanks again. Really Great.
I am so grateful for your post.Really looking forward to read more. Really Great.
Say, you got a nice blog post.Really thank you! Really Great.
This is one awesome blog article.Thanks Again. Great.
I really enjoy the post. Fantastic.
Thanks-a-mundo for the blog post.Thanks Again. Cool.
Thanks again for the article post.Much thanks again. Will read on…
I really enjoy the blog article. Cool.
Really appreciate you sharing this blog.Really thank you! Great.
Thank you ever so for you blog article.Really looking forward to read more. Fantastic.
A round of applause for your blog article.Really thank you! Really Great.
Im obliged for the blog.Thanks Again. Keep writing.
Very informative blog article. Really Cool.
Thanks again for the blog article.Really looking forward to read more. Awesome.
Great blog article. Want more.
I loved your article.Really looking forward to read more. Much obliged.
Hey, thanks for the article.Really looking forward to read more. Really Cool.
Hey, thanks for the blog post. Cool.
Thanks again for the post. Keep writing.
Thanks for sharing, this is a fantastic blog article.Much thanks again. Much obliged.
Thanks a lot for the blog post.Really looking forward to read more. Really Cool.
Wow, great blog article.Really looking forward to read more.
Hey, thanks for the post.Thanks Again. Want more.
Thank you for your blog post.Really looking forward to read more. Really Great.
Thanks so much for the blog post. Really Great.
I really liked your post.Much thanks again. Awesome.
Thanks for sharing, this is a fantastic post.Really looking forward to read more.
Thank you ever so for you article.Really thank you! Will read on…
Wow, great article.Really looking forward to read more. Great.
Muchos Gracias for your post.Really looking forward to read more. Great.
Thanks for sharing, this is a fantastic article post.Really looking forward to read more.
Im obliged for the article post.Really looking forward to read more. Really Great.
I loved your blog article. Fantastic.
Fantastic post.Really looking forward to read more. Keep writing.
Really informative post.Thanks Again. Awesome.
Thanks a lot for the post.Thanks Again. Cool.
I appreciate you sharing this blog.Really thank you! Awesome.
This is one awesome article post.Really thank you! Will read on…
MultiChain
This is one awesome blog article.Thanks Again. Cool.
Enjoyed every bit of your blog article.Thanks Again. Want more.
I am so grateful for your blog. Cool.
Im thankful for the blog article.Really thank you! Cool.
Muchos Gracias for your blog article.Really thank you! Fantastic.
I really like and appreciate your post.Much thanks again. Much obliged.
I think this is a real great blog post.Really looking forward to read more. Much obliged.
Thank you ever so for you blog article. Really Cool.
Major thankies for the blog post.Thanks Again. Fantastic.
Thanks again for the article post.Much thanks again. Really Cool.
Hello, I desire to subscribe for this blog to obtain most recent updates,
therefore where can i do it please help out.
Here is my site :: vpn
sharjah bus going to saif zone
I loved your article post.Really looking forward to read more. Keep writing.
I loved your article post.Thanks Again. Keep writing.
Hey, thanks for the article post.Really thank you! Cool.
I have noticed that credit score improvement activity needs to be conducted with techniques. If not, chances are you’ll find yourself endangering your ranking. In order to reach your goals in fixing to your credit rating you have to make sure that from this time you pay all your monthly expenses promptly in advance of their planned date. It is definitely significant given that by not necessarily accomplishing so, all other actions that you will choose to adopt to improve your credit ranking will not be useful. Thanks for expressing your concepts.
Access to first-rate family medicine in Cool Springs is remarkable. They offer wellness programs with modern facilities and a welcoming atmosphere. Their professionalism makes managing wellness easy for our loved ones.
Im grateful for the article.Much thanks again. Want more.
This fund provides reliable payout income with impressive returns. The experienced handling team delivers consistent value to investors.
I really like and appreciate your article.Thanks Again. Fantastic.